Search Engine Land posted an insightful article today: Apparently Google is featuring images more often in its search results than in the past: https://searchengineland.com/google-starts-showing-more-images-in-the-web-search-results-315804 .
For example: search Google for a keyword, and if Google decides you might be interested in an image search, it’ll show an image bar within the search page. Now this images bar has always existed, but the Search Engine Land article indicates that this bar is becoming more frequent/being added to more searches. Here’s a demonstration:
cupcake
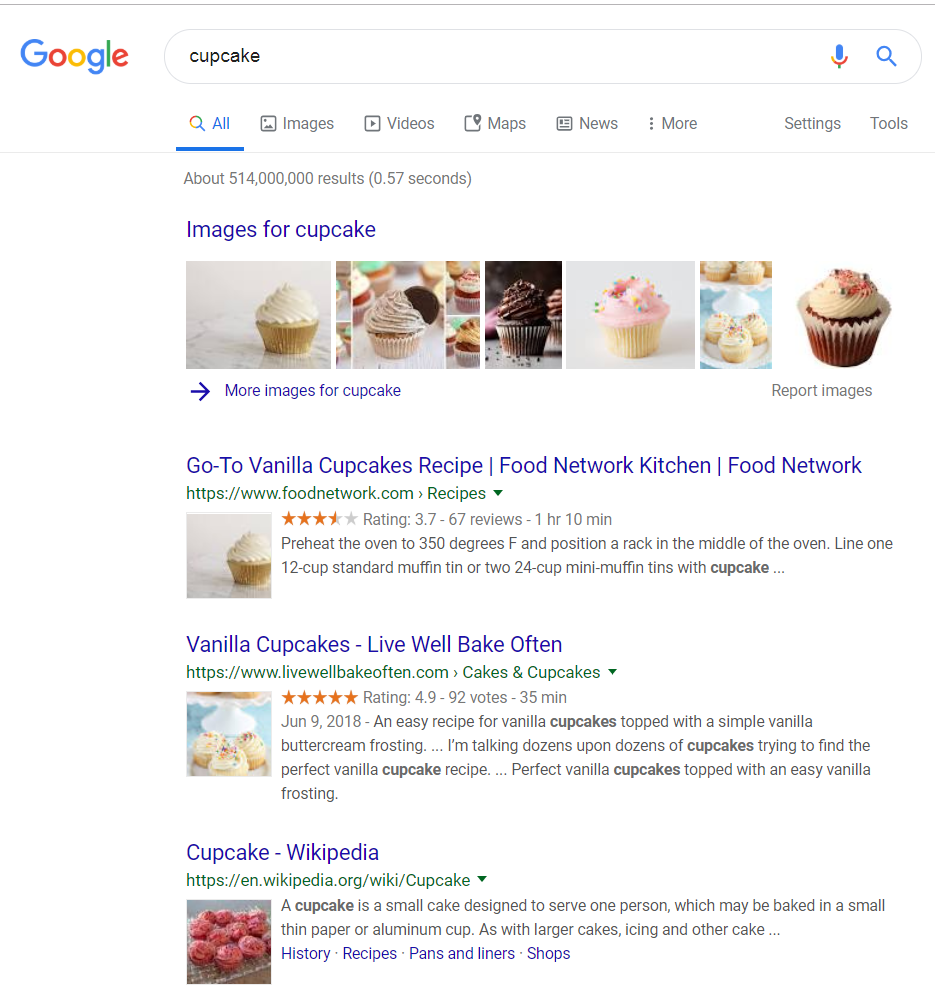
With this new emphasis on images, it’s important to properly SEO images on your website. Make sure to fill out the ALT attribute on the IMG HTML tag, and have a caption explaining the image. Use a high quality image if available.