Integrating Twitter access to your application is fairly simple. But before you write a single line of code, you need OAuth access credentials to log into Twitter. Here’s how to get those credentials.
First, go to https://dev.twitter.com/apps. You’ll see the following screen:
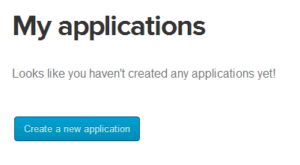
Click on Create Application, then fill in the application details boxes in the next screen:
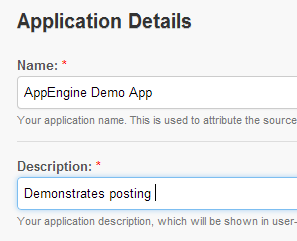
Scroll all the way down and click the button marked Create Application.
After that, go to the Settings tab of your new application:
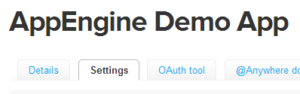
Go down to the Application Type section:
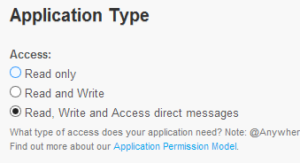
Click on the “Read, Write and Access direct messages” option, and save your settings.
Go back to the details tab:
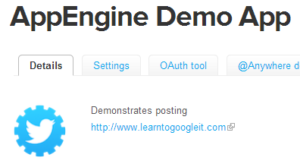
Scroll down and press the button marked “Create my access token”:
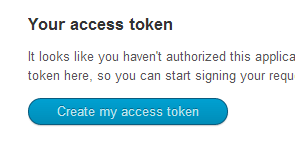
After a moment, you’ll see entries for the OAuth consumer key, consumer secret, access token, and access token secret (they’ll look like long strings of random characters). These tokens can be passed to twitter4j or another Twitter library to log into Twitter.